This documentation will describe the necessary steps to consume the GoTab API in order to create a basic accounting integration.
Fiscal Days
Accounting data in GoTab is attributed to "business fiscal days" or just "fiscal days". An operator using GoTab has the ability to configure their fiscal day schedule according to their business needs. One operator may configure their business fiscal day to be from 12:00AM to 11:59PM. Another operator who stays open later may configure their business fiscal day to be from 2AM to 1:59AM. In the second example the business fiscal day will start on one calendar day and cross over into the following calendar day
In GoTab fiscal days have different statuses. The status that pertains most directly to building an accounting integration is the 'CLOSED' status. A 'CLOSED' status indicates that the business fiscal day is over, GoTab's internal processes have run, and the accounting data is ready to be retrieved. A fiscal day's status is usually updated 'CLOSED' one to two hours after the business fiscal day ends. However, in rare instances, this may not be the case. You may see a 'PENDING' status. For this reason, it is usually a good idea to write retry logic to make an additional request every couple of hours until you get back a 'CLOSED' status and you know the accounting data is ready.
NOTE: real time sales data is available via our GraphQL API, but we highly recommend that, if you can, you use our batch data.
Here is the GraphQL query you should use in order to query a location's fiscal day status:
query FiscalDaysList($filter: FiscalDayFilter) {
fiscalDaysList(filter: $filter) {
fiscalDayBegin
fiscalDayEnd
fiscalDay
fiscalDayStatus
}
}
variables:
{
"filter": {
"fiscalDay": {
"equalTo": null
},
"locationId": {
"equalTo": null
}
}
}
The Ledger
Once the fiscal day is closed you will want to pull that fiscal day's data from our ledger.
GoTab maintains a ledger, which is a flat data structure that contains all of the data that affects a location's profit and loss. It is designed to be accurate and easy to work with. This is the same data that we use for our internal reporting so the goal should be reconciliation between the GoTab system and yours without discrepancies.
There is a plethora of data available on the ledger, but here is an example query that will return enough data to implement a basic accounting integration:
query LedgerEntriesList($filter: LedgerEntryFilter, $first: Int, $offset: Int) {
ledgerEntriesList(filter: $filter, first: $first, offset: $offset) {
amount
fiscalDay
transactionName
transactionTime
tabUserId
orderUserId
accountingStream {
name
reportingGroup
}
tabLocationId
propertyLocationId
product {
productId
}
}
}
variables:
{
"filter": {
"fiscalDay": {
"equalTo": null
},
"propertyLocationId": {
"equalTo": null
},
"tabLocationId": {
"equalTo": null
}
},
"first": null,
"offset": null
}
Working With the Data
The first thing worth looking at for an accounting integration is how we aggregate our data for reporting on our sales page (image below). In GoTab products are mapped to an "account" or "accounting stream" and each account is mapped to an "accounting group." There are some default accounts that we create for each location, but an operator can and often will configure their own. Each reporting group (reportingGroup in the above query) is an enumerated, default value that is created by GoTab.
Here are the values that you may see for reportingGroup: NET SALES, AUTOGRAT, TAX, DEFERRED REVENUE, RECEIVABLES, TIPS, FEES, CHARGEBACKS, PROCESSORS, OTHER, EXPENSE.
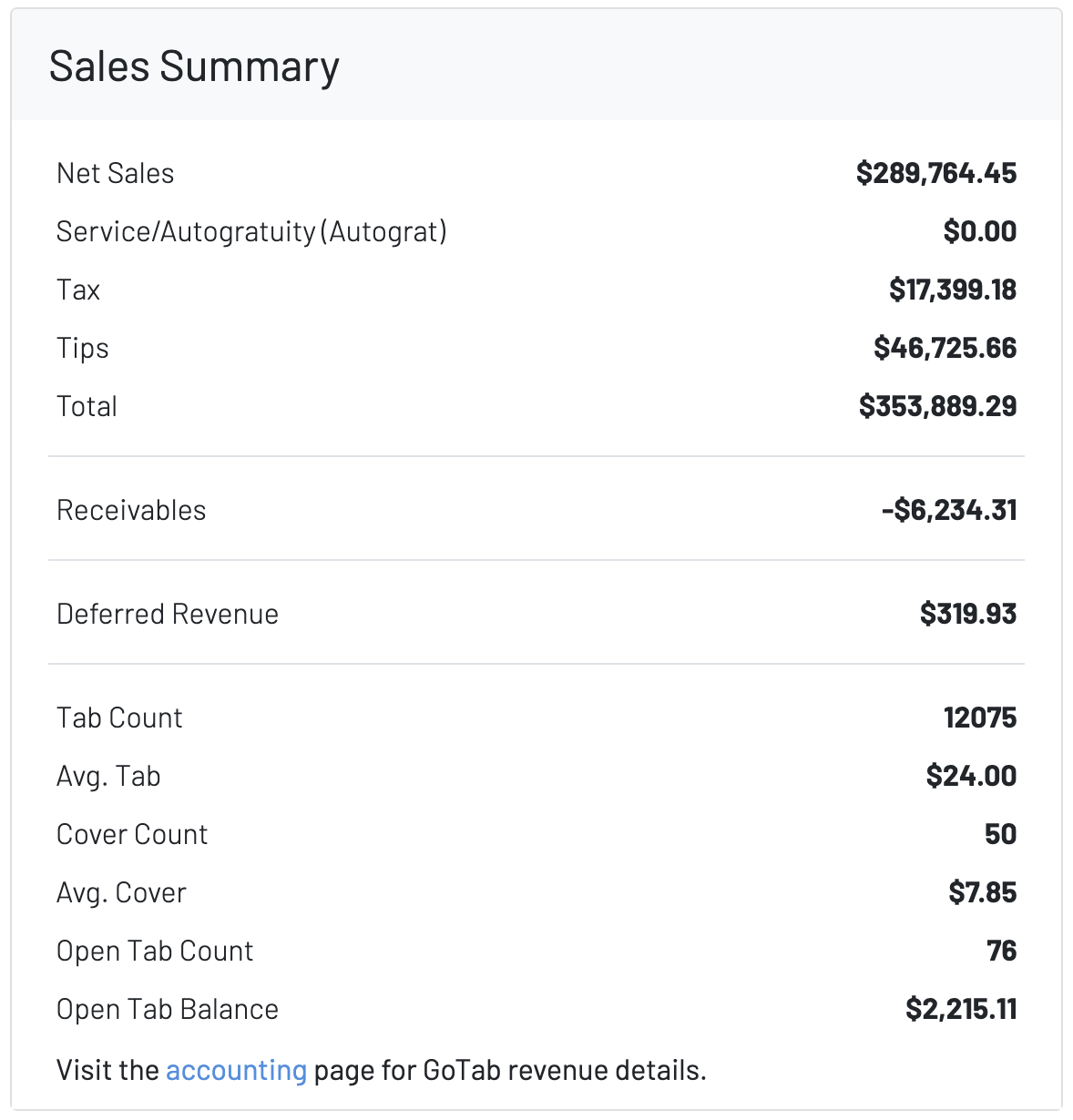